What is ASP.NET MVC and Web API?
- MVC framework that uses C# and IIS as the programming language and web server
- Web API allows for simple creation of REST APIs
- Razor templates to implement a combination of HTML and C# using cshtml files
- Integrates easily with frameworks like Entity Framework to simplify the integration to databased like MSSQL and MySQL
- Built-in login system using ASP.NET Identity membership system including social logins such as Facebook and Twitter
- Easily add external libraries like JavaScript Fetch API
ASP.NET MVC and Web API Examples using C#
Below are a list of MVC articles that will provide the essential tools to take you from a beginner to an expert with MVC by providing clear and concise demos with practical, real-world examples. Whether you are looking for beginner tutorials or advanced tutorials these examples will provide the essentials to become more proficient with the MVC framework. These examples have a mix for C# and SQL server so don't miss out on great articles that use SQL Server Convert String to Date with examples.
The example below will create an array, if you're looking for information about JavaScript arrays I've compile an entire page dedicated with over 10 different examples that will considerably improve your skills with Javascript arrays.
When you think of C# and web automation, your mind might first leap to Selenium. But what if I told you there's a headless browser automation tool with a slick, modern API, powered by the Chrome DevTools Protocol, and it's *blazingly fast*? Enter: PuppeteerSharp.
C# is a powerful and feature-rich programming language that offers various constructs to enhance code readability, performance, and maintainability. One such construct is the `yield` keyword, which provides a convenient way to implement lazy evaluation. In this article, we will explore the `yield` keyword in C# and learn how it can simplify your code while improving its efficiency.
When working with dependency injection in C#, you'll often come across three common methods for registering services: `AddTransient`, `AddScoped`, and `AddSingleton`. These methods are used to configure the lifetimes of services within your application. In this article, we'll explore the differences between these three methods and when to use each of them.
Microsoft Excel is a widely used spreadsheet program for creating, manipulating, and analyzing data. However, to work with Excel files in C#, traditionally, you would need to install Microsoft Office or rely on third-party libraries. In this article, we will explore an alternative approach to generate Excel files (.XLS and .XLSX) using C# without the need for Office installation. We will leverage the EPPlus library, a powerful open-source library for working with Excel files in .NET.
Email communication plays a vital role in our digital lives, and as a .NET developer, it's essential to know how to send emails programmatically. In this article, we will explore how to send emails in .NET using the Gmail SMTP server.
Gmail provides a powerful and reliable email service that can be easily integrated into .NET applications. To send email through Gmail, we will leverage the System.Net.Mail namespace in .NET, which provides classes for creating and sending email messages.
If you've received the following error: Specflow all steps have been defined in this file already then you've come to the right place.
An exception of type 'System.ArgumentOutOfRangeException' occurred in mscorlib.dll but was not handled in user code. Let me show you how to solve it.
This article covers sp getapplock / releaselock storage procedures and its use in a simple way including simple functions that can be used with Entity Framework and C#.
Leveraging C#'s substring to truncate a string, I am going to demonstrate how to create a string extension with a new re-usable function called Truncate.
In these examples, I will demonstrate how to convert a string to boolean and an integer to boolean using C#.
Entity Framework Core allows users to execute raw SQL queries directly against the database. Let me show you how.
In this tutorial, I'll show you how to implement basic authentication using ASP.NET MVC implementing the AuthorizationFilterAttribute Filter
The CORS (Cross Origin Resource Sharing) ASP.NET Web API allows us to request data from another website without having to use JavaScript.
To return a CSV file using C# you need to create a new StreamWriter and add the CSV formatter to your Web API. I have a full example project to demonstrate.
Let me show you how to use Enum.GetValues to convert an Enum to a List using C# with practical examples.
Let me show you how to easily Catch multiple exceptions with C# through multiple examples inside a single try block.
Let me show you how to use Entity Framework to update only changed fields when doing a SaveChanges with a handy re-usable function.
Let me demonstrate how to use C#'s TimeZoneInfo.ConvertTimeFromUtc and TimeZoneInfo.ConvertTimeToUtc to convert from UTC to EST or EST to UTC
Let's learn all about what MVVM is through practical examples.
The following extension method is probably one of my all time favorite things to do with strings: parse out text between a start and end delimiter.
When you have a single web server, storing the user sessions on the single server works perfect; however, when you have multiple web servers and users need to potentially round robin between servers or you need to remove servers from the pool and don't want to affect your user's experience enter: SQL Session State.
When I first started working with IIS again after working with PHP for so long, I struggled to accept the 20-30 second load time when the website's AppPool is not running. Here is a nice quick little fix to keep your important websites always running.
Let me show you a quick method to convert Camel Case in C# to title case.
So many times to have a list of objects that I want to group - as an example - by a customer ID. The end result that I would like would be a: Dictionary<long, List>
To generate a random number it involves instantiating the Random class then calling the Next function with a minimum and maximum value as follows: new Random().Next(min, max) so why is it always returning the same number? This commonly occurs when this is being called in a loop.
I like when integrations like Twilio are simple and straightforward. I recently had the privilege of implementing it for my company. For my implementation I have a couple of special requirements that I think makes my solution better than just referencing a NuGet package and calling MessageResource.Create.
My requirements require creating user controlled text messaging templates and customer controlled enabling/disabling of one or more text messages. Let's get started.
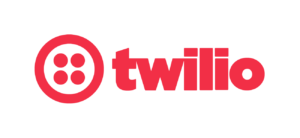
Like many requested features, MVC 5 now has a built-in function to provide an Enum object and automatically build a dropdown list using the standard HtmlHelper. If you're unfortunate and on an older version of MVC, here is an HtmlExtension that performs the same thing.
The JSON request was too large to be deserialized is a very common error because of MVC's default size for a JSON response from the Web Api. Luckily there is a very simple solution that requires a minor change in your Web.config
There has been a lot of kerfuffle over Chrome's upcoming change to how cookies are based when one website is iFraming another website in an effort to further improve the security of the Internet.
At the end of the day, the solution is to set your cookies - specifically the .ASPXAUTH cookie - so that when users navigate the website of the iFrame source the cookies will be passed from page-to-page. This is very important to those who are using FormAuthentication.
The solution requires two changes. Let's look at them now.
Cloning is a common thing I need to do in my C# project. The most common case I use this is with my Entity Framework projects. I first fetch my object using a standard Linq query.
Once I have this object, EF is now tracking any changes to the object. I want a duplicate object of this so I can track a before and after state, so I want to clone the fetched object. To do this I use JSON.Net nuget package.
Short answer: nothing. string is an alias to System.String. This also holds true for int and System.Int32.
If you want my personal preference I always use the lowercase version aka the alias. Why? Because I don't need to add the following reference: using System; in each file. I know, it's a little OCDish, but what can I say...
Web API and MVC with C# offer stellar global filters that can be registered in the App_Start/FilterConfig.cs file. For Web API and MVC the global filters goes inside the RegisterGlobalFilters function. This function contains an override based on whether you are applying the filter to MVC or Web API. Today we are going to look at how to implement an extension filter of the ExceptionFilterAttribute.
I was struggling the other day where one of my NuGet packages DLL that was installed in a separate MVC project was not being copied to my bin directory. I have discovered two potentially reasons why this was occurring. Read on for two different potential solutions.
Recently I was stuck with the error Try removing one of the references or sign them to enable side-by-side. This is not a common error, but I received it because I was in the very unfortunate situation of requiring to have two different versions of the same DLL installed in my solution. Let's explore how I worked around this.
I recently showed how to setup an SSL certificate using nginx, in this article I will demonstrate how to create a Self-Signed SSL certificate that can be used with IIS.
Let's create an HTML Extension for C#'s HtmlHelper class that converts a class or object to JSON for use with your JavaScript code. I use this in my MVC projects inside my Razor Views. The solution leverages NewtonSoft's JsonConvert NuGet package to convert with C# HTML to JSON.
I use Ninjection for my C# MVC project. As I've discussed in Advanced Automatic Ninject Bindings Advanced my Ninjection is automated based on Interfaces matching Class Names. So when the dreaded Controller does not have a default constructor error occurs I have two common starting points.
Let's explore creating an extension method to convert a number to an ordinal value, e.g. 1st, 2nd, 3rd, etc.
If the following blog interests you, it's probably because you're using Entity Framework with a Code-First approach and whatever it is you are trying to do *must* use an ObjectContext instead of the standard DbContext. I'm sure, like me, you thought this shouldn't be too hard. Then you started hitting roadblocks like, Metadata is required in your connection string. What's metadata? This is only needed in Database-First so you can tell the framework where your edmx and other definition files are, so why do I need this with Code-First, I don't have these files?
This was definitely my first reaction as well. So after much trial-and-error and research, I have the solution!
In a lot of the MVC 3 examples that are available on the Internet today, they are quite typically strongly typed to a model, e.g. public ActionResult LogOn(LogOnModel model, string returnUrl).
This is extremely useful for the validation abilities and many other aspects; however, there are times when some or all of the data is not strongly typed; then what do you do? Read on for two different approaches.
Have you ever wanted to pass form data or perhaps even a full model from one action to another through a RedirectToAction? By adding a new library package from NuGet, this can be accomplished with a few small changes to your controllers.
The first thing that needs to be done is to install the package through the NuGet package manager. Inside of Visual Studio, with the your MVC project selected select Tools -> Library Package Manager -> Add Library Package Reference… On the left hand side, select the Online button. Then, in the search field, type MvcContrib and install the base package and you can explore how to truncate string with C#.
When you have a website with a lot of static content: images, Javascript, CSS, etc… you will find yourself constantly typing out the same path information with the exception of the name of the actual file. Creating a class extension to the UrlHelper can help greatly reduce your development efforts with a few simple additions.
Most ASP.NET developers will use Visual Studio to build their projects. The program has evolved quite a bit over the past few years. Including excellent features like Intellisense inside of ASP.NET MVC view files as well as some error detection in these, by default, not compiled elements. However, when ViewBag variables are used or other run-time specific elements, Visual Studio is unable to determine potential errors in these view files. Have no fear though; there is a nice and simple solution to help solve this problem!
You want to perform some dynamic processing in your code and you need to determine either the name of the current controller or the current action or both. Enter the object ControllerContext.RouteData.
You have SVGs that you wish to display on your website but you want to be able to re-use them without copying and pasting the code or creating a shared view.
By creating an HtmlExtension class with a function called Svg you can create a centralized place to store and re-use your SVGs.
I often find myself using this great C# function: string.IsNullOrEmpty. It is the assured way to confirm whether a string is empty - null or otherwise. However, I often find myself wanting to know when it is not empty, so I wrote an string extension named HasValue.
The other day I was working on a project that was leveraging OutputCache. This little attribute is a fantastic way to implement caching in an MVC project. This was working great until I encountered a snag when using a subdomain.
You've added some data validation and you want to test it out in action. The data validation can be standard data annotations, custom data attributes, or data validation implemented via an IValidatableObject. This article will explore how to leverage the ValidationContext to execute and assert the test results.
You require custom data validation that cannot be accomplished via the built-in data annotation validation attributes - or - your data validation requires access to multiple properties in your model. Let's explore how to leverage the IValidatableObject.
Please note this is an excerpt from Chapter 9 of my ASP.NET MVC 5 with Bootstrap and Knockout.js book. In this chapter I provide a brief overview of the 5 different MVC filters and then over the next two chapters provide detail examples of each. The following post is leveraging the example of a Result Filter.
I can't believe 9 months has gone by since I came to an agreement on writing two books with O'Reilly Media! The first book was on Knockout.js which is a great framework that focuses on the Model-View-ViewModel (MVVM) architecture pattern. I finished the initial draft at the end of September 2014. During October and November, I multi-tasked by writing the second book while working through copy edits and multiple rounds of QC on the first book.
The concept for this book started with creating many Knockout js tutorials and the first book official released (in e-book format) in December with the print version releasing early in January.
December, January, and February were all busy months while I was working on the second book. I cannot stress how difficult this book was to write. Each chapter would take days to write, tweak, and finalize all the while continuing to write blogs like how to use SQL Server Convert String to Date with examples.
March, and now April contained more copy edits and QC rounds for the second book. I think the book is about to be finalized and the e-book version should be ready sometime in the middle of May with the print copy following shortly after!
As the title of this blog states, the book is titled ASP.NET MVC 5 with Bootstrap and Knockout.js. The (un)official back cover reads as follows:
"Bring dynamic server-side web content and responsive web design together to build websites that work and display well on any resolution, desktop or mobile. With this practical book, you’ll learn how by combining the ASP.NET MVC server-side language, the Bootstrap front-end framework, and Knockout.js—the JavaScript implementation of the Model-View-ViewModel pattern.
Author Jamie Munro introduces these and other related technologies by having you work with sophisticated web forms. By the end of the book, experienced and aspiring web developers alike will learn how to build a complete shopping cart that demonstrates how these technologies interact with each other in a sleek, dynamic, and responsive web application.
- Build well-organized, easy-to-maintain web applications by letting ASP.NET MVC 5, Bootstrap, and Knockout.js do the heavy lifting
- Use ASP.NET MVC 5 to build server-side web applications, interact with a database, and dynamically render HTML
- Create sleek and responsive views with Bootstrap that render on a variety of modern devices; you may never code with CSS again
- Add Knockout.js to enhance responsive web design with snappy client-side interactions driven by your server-side web application"
I think this does a great job of describing why I chose these three technologies for the book and how they come together allowing you to easily build dynamic and responsive websites.
While writing these two books I jotted down a lot of ideas for examples. Unfortunately (or fortunately), I was unable to include them all into the books. So over the next little while, I will work to bring them as examples on my blog. Stay tuned. If you have any questions about the books, feel free to post questions/comments here on my blog or find me on Twitter @endyourif
I hope this isn’t too extremely obvious, but I found that I had to take a step back and re-examine my unit tests to find this simple improvement to speed up my unit tests.
When I wrote about how I’m hooked on test-driven development (TDD), the example in that post was too simple and time savings are not noticed. However, let’s dive in to something a little deeper where we have a full class to test oppose to a single internal function.
You have a common interface that is used by multiple concrete classes and you don’t want to specify each Ninject binding manually.
You have a large project with many interfaces and many different concrete implementations. Because of this, managing every single Ninject binding is becoming challenging and time consuming.
By leveraging an additional Ninject NuGet package called Ninject.extensions.conventions, you can write a single line (wrapped over several for readability ;) that will manage all of your Ninject bindings.
I’ve only been doing TDD for a few weeks, but I’m completely sold. I don’t want to go back! I’ll be honest though, it hasn’t been easy. I’ve made mistakes, I’ve wasted time, but I’m really starting to reap the benefits.
I’ve always thought I was a good developer. I write decent code and it works mostly as expected. It took me many years into my career before I wrote my first unit test. It always fell into the category of too time consuming or expensive. Oh the irony!
As I started learning how to write to unit tests, I always found myself rewriting things I already did just to get them to be unit tested; how frustrating! A unit test that should have only took a few minutes, ended up taking a really long time because the code had to be refactored just to be tested. No better way to turn you off from unit testing.
Enter test-driven development…
I hate typing more lines of code then I need to; especially something as simple as mapping a domain model to a view model. Enter Automapper!
By performing a one-liner:
Mapper.Map<Customer, CustomerViewItem>(customer);
I can quickly map my domain models to my view models.
I was recently reviewing an old article on CodeProject: http://www.codeproject.com/Articles/61629/AutoMapper that contains a quick and easy demo. Inside this article, it discusses performance and it indicates that Automapper is 7 times slower than manual mapping. This test was done on 100,000 records and I must say I was shocked.
My first thought is this requires more testing. Especially since 100,000 records is a lot. In most scenarios I would estimate my largest mapping might be 1,000, but even 1 would probably be a very regular use-case. Let’s put it to the test…
After the incredible reaction to a recent blog post, Entity Framework Beginner’s Guide Done Right, I feel like before writing some more code to further the basic example, I’ll take a step back and explain my beliefs in the repository pattern.
I was really overwhelmed with the reaction; what started with a simple 30 minute blogging effort has turned into something absolutely incredible. The original post was really a starting point about not placing direct querying and saving to the database mingled with the core code.
Please note, these are my thoughts on the pattern based on current and previous pain points that I’ve/am experiencing and I’d love to hear others input in making things better.
Entity framework is a great ORM provided by Microsoft. There are a ton of examples of how to get up and running with it really quickly. The only problem with all of them, is the get you off on the wrong foot.
In all of the EF example guides, the DbContext class is typically deeply embedded into the core of your code. This of course is great for Entity framework because the effort to change will be next to impossible – speaking from experience of course here.
Instead, by making some subtle changes we can integrate Entity framework in a separate layer in case at some later date you wish to replace it. Of course, you might never need to replace it, but following these simple techniques will allow better segregation of code and even provide simpler unit testing.
I recently had the pleasure of some free time recently – with three kids this does not come often – so I decided to finally plug my Kinect into my PC. I find it kind of funny how long it actually took for this to happen. Over a year ago I finally joined the real world and made the decision to finally make a new console gaming purchase.
Like everyone, I had to choose between the PS3 and Xbox 360. Each of course has their pros and cons. But when it came down to it, I could develop for the Xbox and Kinect for free and this became the deciding decision factor.
So finally over a year later I finally plugged my Kinect into my PC’s USB port!
I recently had the opportunity to begin exploring and toying around with Windows Phone 8 development.
Why you ask? Why not really, but mostly because I was giving a free phone and it’s an untapped market when it comes to apps. At this stage it’s not over diluted like the Android and iPhone stores are. That and of course there is an option to create apps using HTML, Javascript, and CSS – technologies I’ve used and mastered for years!
I actually submitted my first app last weekend – and sadly – it got rejected :( I was given two reasons; firstly I did set a default application icon, whoops my bad. Secondly, I didn’t properly handle the back button since there are “multiple pages” in my application.
It took me several hours to finally found the answer so I thought I would share it. Please note, this feels like a bit of a hack, but I wasn’t able to get the “suggested” solutions working…
If you use a framework of some sort, you probably haven't thought about SQL injection for some time – in fact it almost seems dated to even discuss it. However, security should never be overlooked and it's important to not trust third party applications and people by default! So what is the best way to prevent SQL injection?
Have you noticed how I haven't specified a specific language? This is done purposely, because at the end of the day – all languages – should be able to follow this paradigm…
Well – it's Friday and all of the kids are back in school. While this post is being published, I'm probably stuck in traffic! I can't believe it's a new school year already, luckily my kids aren't old enough so it's just traffic that I need to get used to.
This has been a great summer so far and I thought it would be a good idea to summarize the variety of things I've learned about – but have not necessarily blogged about…
A little while ago, I wrote a simple .NET application that performs X amount of requests and calculates an average speed of those requests. It does this by dropping the highest and lowest request times, then taking an average speed on the remaining requests.
This does a decent job for a straight up speed test. However, a few possibilities could arise, such as CPU hogging that could skew the results. Instead, I've made a few alterations and converted the speed tester to not be based on the number of requests, but instead based on a specific amount of time. This should help eliminate some inconsistencies of doing a straight number of requests.
Below is an excerpt of a chapter from my new book: 20 Recipes for Programming MVC 3.
In today’s heavily fought battles for search engine supremacy, it’s quite difficult to winthe race with a website address that looks like:
http://www.example.com/books/details?id=4.
Using routes, the website can look like:
http://www.example.com/20-recipes-for-mvc3
which provides much more context, both to the user and the search engine.
Yesterday marks the official launch of my second book: 20 Recipes for Programming MVC 3. The book is being published by O'Reilly and is available in both e-book or print edition. I'm quite proud of this book as I've feel like my writing style was really able to mature while working with a real editor to bring a great cookbook on ASP.NET's MVC 3.
The tagline for the book is, Faster, Smarter Web Development and that truly is my goal with all of the recipes in the book. It covers basic material from authentication and emails to more complex features like routing and AJAX - even has an excellent tutorial on converting your website into a mobile site in a few easy steps!
I have recently being working on my largest multi-lingual site ever - over 30 languages - in ASP.NET. While implementing it, there have been a lot of bumps and bruises along the way. One of the most recent one was noticing that the ToUpper and ToLower functions in ASP.NET take the CurrentCulture.CultureInfo into consideration.
As you may guess, this is both a blessing and a curse.
When I'm working with text that is to be translated, but should appear upper or lower case this makes perfect sense that the CurrentCulture.CultureInfo should be taking into account. However, be sure you remember this later when you are doing text transformations and text comparisons on words that should NOT be translated (like when using convert date with C#).
At the end of the day, if you don't want the CurrentCulture.CultureInfo to be used, both functions can be easily overloaded as follows:
String.ToUpper(new CultureInfo("en"))String.ToLower(new CultureInfo("en"))
I spent a fair bit of time tracking down why existing code was working perfectly, when suddenly as the team implemented the 8th language, something suddenly started failing. Thank goodness for unit testing to quickly point out that there was a problem - then it was just a matter of understanding it!
ASP.NET MVC and Web API Book
If you're looking for or a PDF version of ASP.NET MVC I have written a book called Asp.Net Mvc 5 with Bootstrap and Knockout.js: Building Dynamic, Responsive Web Applications. This book starts at the beginning and takes you write through building a nice shopping cart application with many re-usable pieces of code.